What is ReactJs?
As the official ReactJs docs say, ReactJs is nothing but ..A JavaScript Library for building User Interfaces
We will look at things one by one that ReactJs offers to better understand why it is the most loved library for web.
Component
React uses Component Based architecture. The whole UI can be de-composed into small, re-usable components.
A component is an independent, reusable code block.
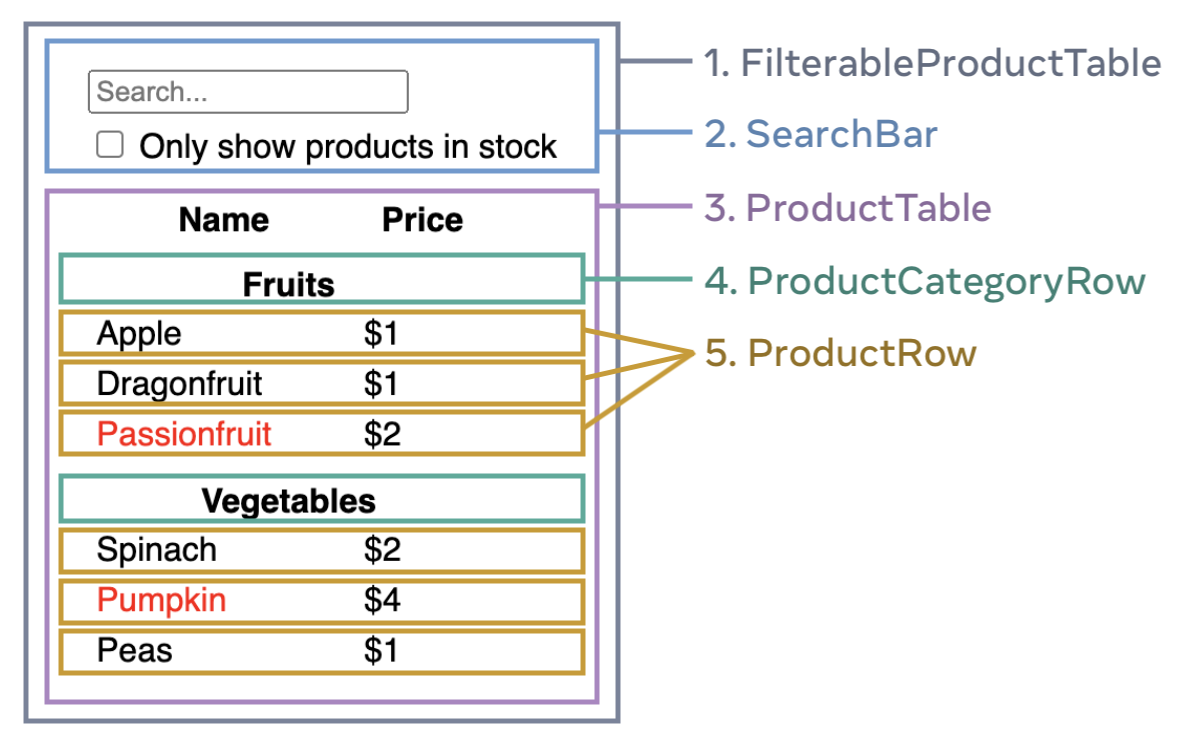
As you can see in the image, the UI is divided into multiple small, re-usable components.
The ProductRow
component is rendered differently by passing data via props. Okay, but what are Props? Don't worry.. we will get to it.
The FilterableProductTable
is the Parent component of two Child components SearchBar
and ProductTable
.
Data (state) can be shared between components. Parent to Child (via props), Child to Parent. (pass function prop. Child calls that using data)
Virtua DOM
Virtual DOM is the virtual representation of Real DOM. React update the state changes in Virtual DOM first and then it syncs with Real DOM.
Virtual DOM - Virtual Document Object Model
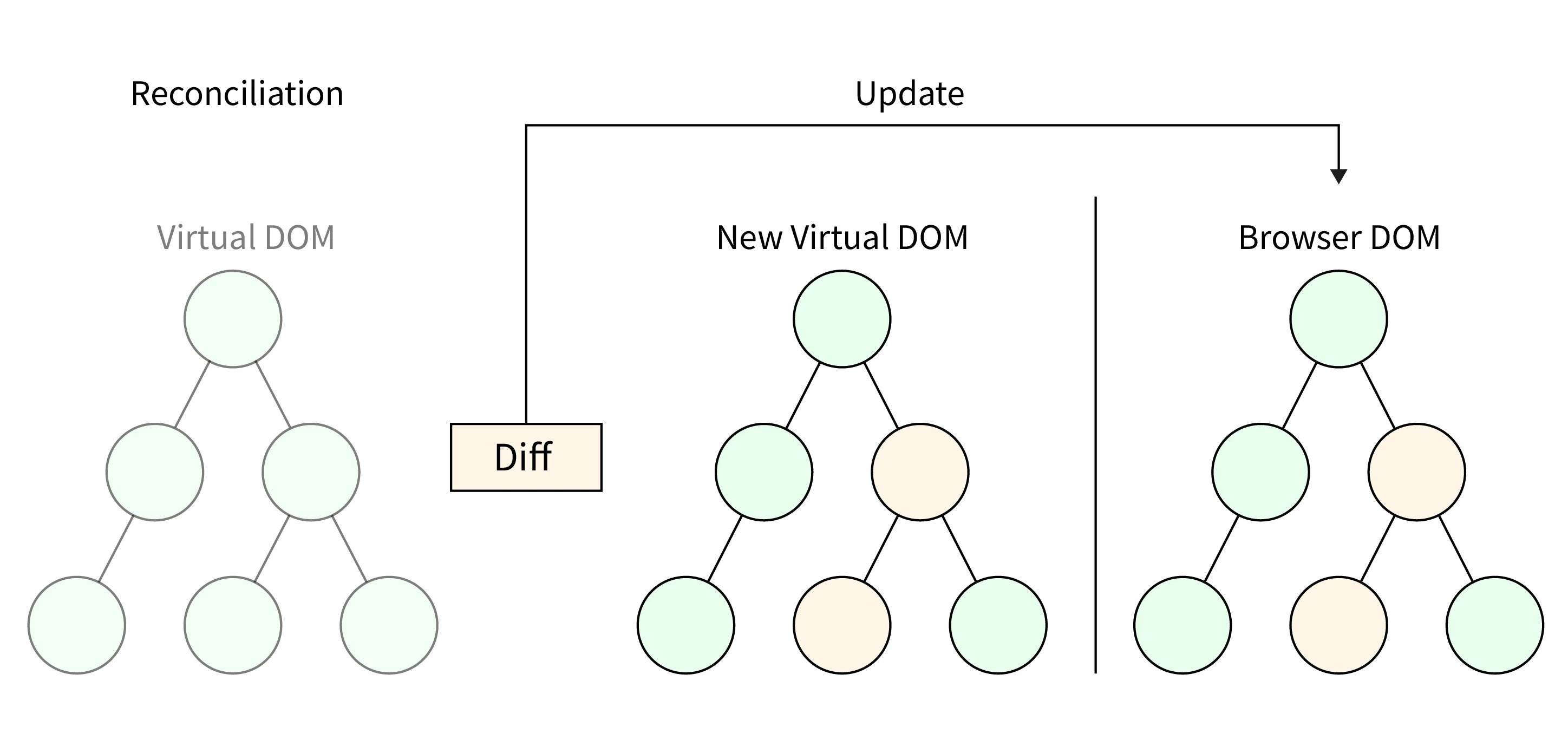
Runs diffing algorithm to compare the previous created component tree with the new one and does Reconciliation.
Props and State
Props (Properties) is data which is passed from Parent to Child. State is maintained by the component itself. Component re-renders if State or Props changes.
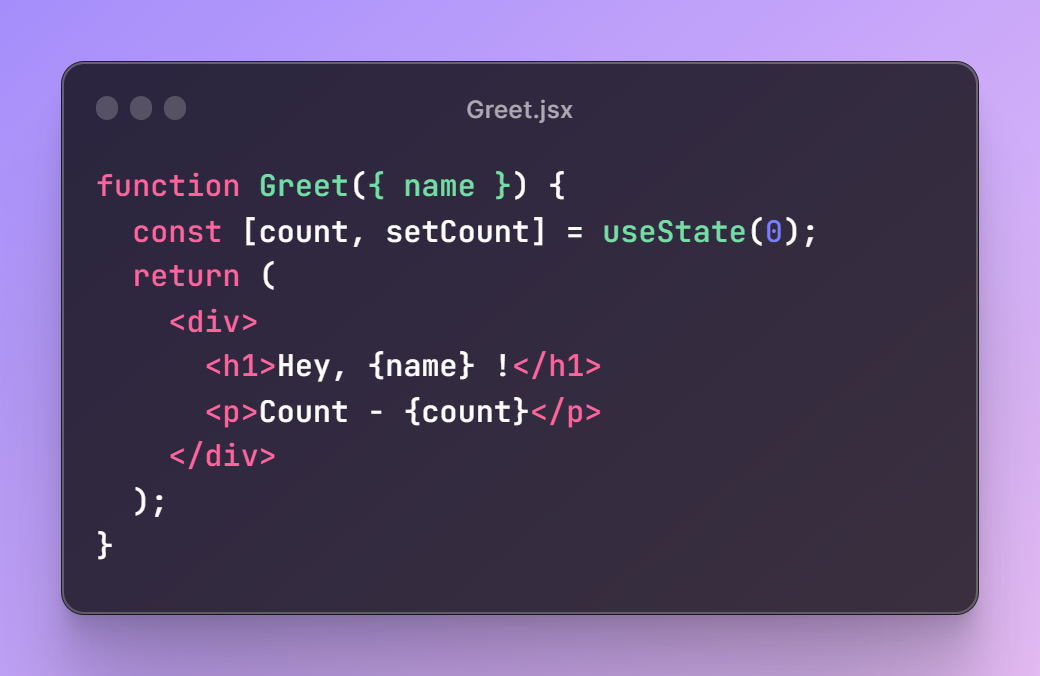
Okay. That's lot. Lets understand. (useState
- more on this later)
Greet is Functional component (ReactJs has Class components too). HTML-like markup inside a JavaScript function is JSX. (syntax extension for JavaScript)
It receives name
as prop from it's Parent and renders it.
count
is state, which is maintained in the component itself. setCount
function is used to manipulate count
.
Component is written using JSX, Syntax extension to JavaScript. Most React projects use JSX for its convenience. It makes defining components easier.
Hooks
Hooks let you use different React features from your components.
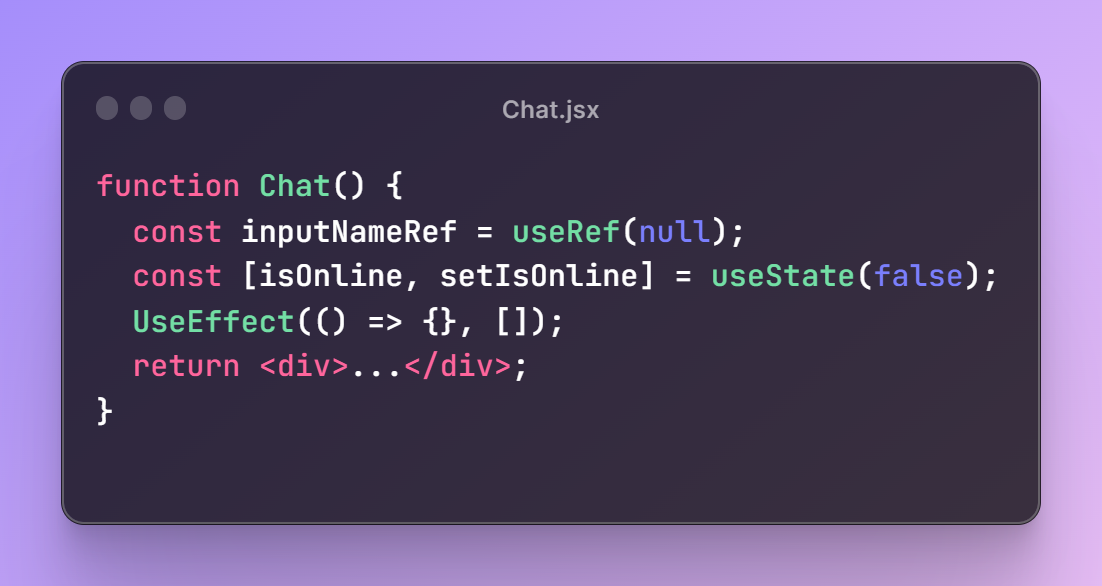
Few important hooks include..
useState
- State lets a component remember information. (string, number, object etc) useState
takes initial state value as argument. isOnline
is the state. setIsOnline
lets you manipulate it.
useRef
- Hook that lets you reference a value that’s not needed for rendering. (timeoutId)
useEffect
- Effects let to perform actions like fetching, state manipultion etc based on the dependencies you provide.
useContext
- Lets you read and subscribe to context(state) from your Component.
useFetch
- We can build our own custom hooks like this one. This hook lets you make fetch calls with ease by customization.