How NodeJs works?
Firstly, What is NodeJs?NodeJs is a JavaScript runtime environment.
Traditionally we used to run JavaScript only in the browser (because they have JavaScript engines). Thanks to NodeJs we can run JavaScript anywhere.
We can build HTTP servers using NodeJs. Visit NodeJs and download Node.
Go to terminal and run the command node -v
. If Node is succesfully installed, you will see the version.
Basics
Create a index.js
file and write the below code.
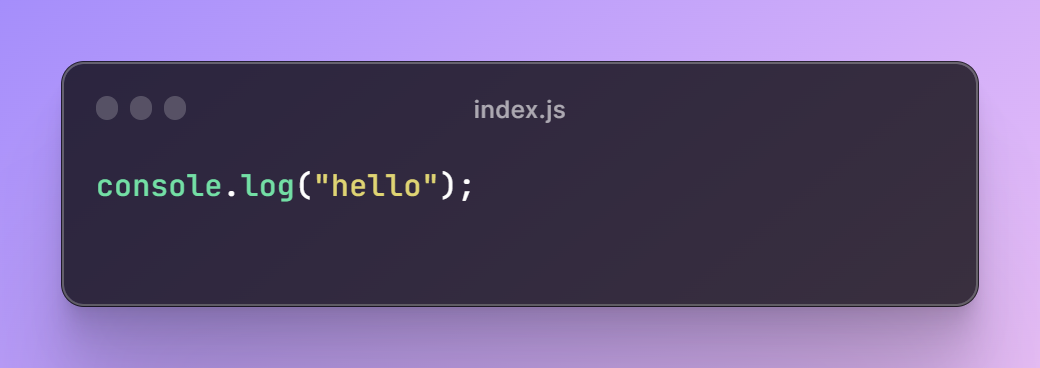
If you run the command node index.js
, it prints hello
on the terminal.log()
is a built-in method which lets you print to the console.
File I/O
Using built-in module fs
provided by Node, we can manipulate file system on the server.
NodeJs for manipulating files on the server
Create a tmp.txt
and place hello world !
in it. Now place the below code in index.js
.
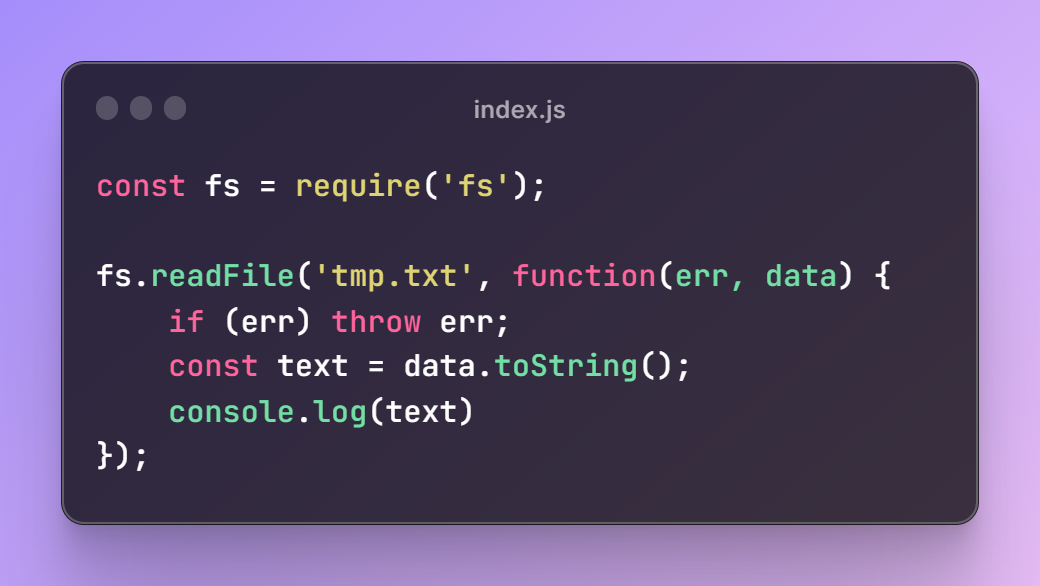
If you run the program, you will see hello world !
on the console.
Import fs
module (built-in). The readFile
method takes the file path and callback. The callback will be executed when the file is done being read.
The data will be in Buffer
. We have to convert it to string using toString()
method.
We can write/ append to files. We can even remove files by using fs
module.
HTTP Server
NodeJs as HTTP Server - Serve static files or build API endpoints for various purposes.
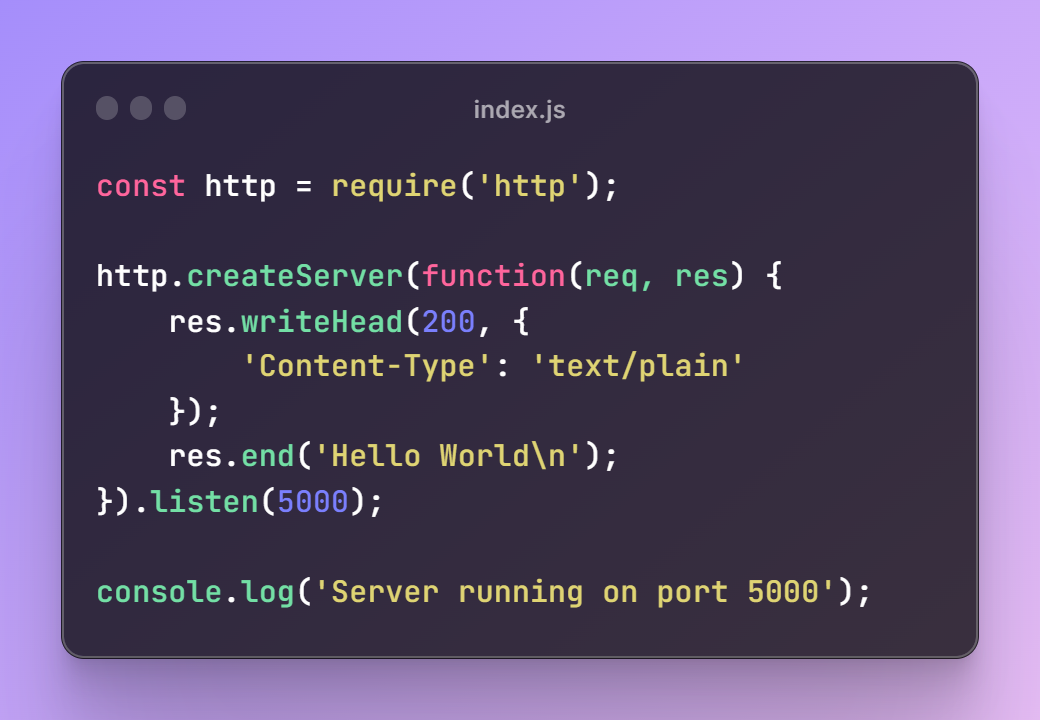
http
module is used for creating http server. If you hit the localhost port 5000
, you will receive the data Hello World
.
Import http
module. The method createServer
takes in a callback function. The req
and res
are Request and Response objects.
Request object contains the URL (path params, query params etc), body (json, blob etc), headers etc. We can append data to Response object that we want to send to the requester.
writeHead
method lets you set headers. end
method lets you end the current response process after sending (optional) data in it.
Using listen
method we can set the port that we want our application to run.